import matplotlib . pyplot as plt import numpy as np 关键字参数的设置形式 def no1 (): """ 关键字参数的设置形式 :return: """ fig = plt . figure () ax = fig . add_subplot ( 111 ) font = { "family" : "serif" , "color" : "na
import numpy as np
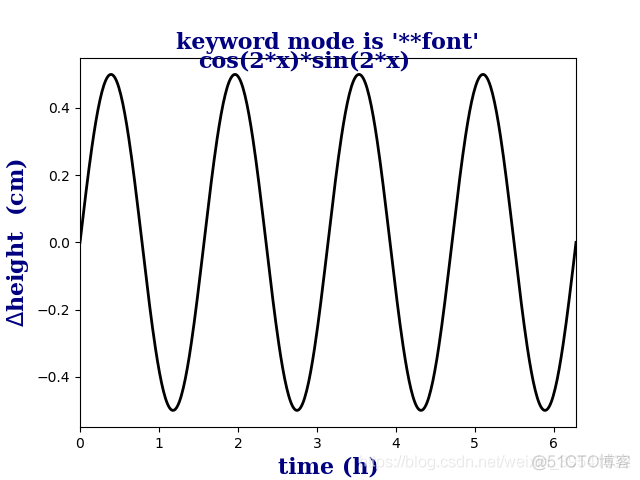
"""
关键字参数的设置形式
:return:
"""
fig = plt.figure()
ax = fig.add_subplot(111)
font = {"family": "serif", "color": "navy", "weight": "black", "size": 16}
x = np.linspace(0.0, 2 * np.pi, 500)
y = np.cos(2 * x) * np.sin(2 * x)
ax.plot(x, y, color='k', ls='-', lw=2)
ax.set_title("keyword mode is '**font'", **font)
ax.text(1.5, 0.52, "cos(2*x)*sin(2*x)", **font)
ax.set_xlabel("time (h)", **font)
ax.set_ylabel(r"$\Delta$height (cm)", **font)
ax.set_xlim(0, 2 * np.pi)
plt.savefig(r"E:\Programmer\PYTHON\Matplotlib实践\figure\Figure(Unit "
r"9)\no1.png")
plt.show()
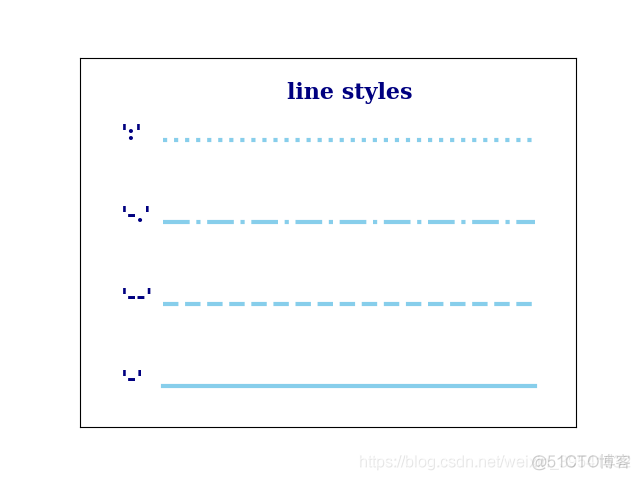
"""
线条类型的显示样式设置方法
:return:
"""
font = dict(family="serif", color="navy", weight="black", size=16)
color = "skyblue"
linewidth = 3
fig = plt.figure()
ax = fig.add_subplot(111)
linestyleList = ['-', '--', '-.', ':']
x = np.arange(1, 11, 1)
y = np.linspace(1, 1, 10)
ax.text(4, 4.0, "line styles", **font)
for i, ls in enumerate(linestyleList):
ax.text(0, i + 0.5, "'{}'".format(ls), **font)
ax.plot(
x,
(i + 0.5) * y,
linestyle=ls,
color=color,
linewidth=linewidth)
ax.set_xlim(-1, 11)
ax.set_ylim(0, 4.5)
ax.margins(0.2)
ax.set_xticks([])
ax.set_yticks([])
plt.savefig(r"E:\Programmer\PYTHON\Matplotlib实践\figure\Figure(Unit "
r"9)\no2.png")
plt.show()
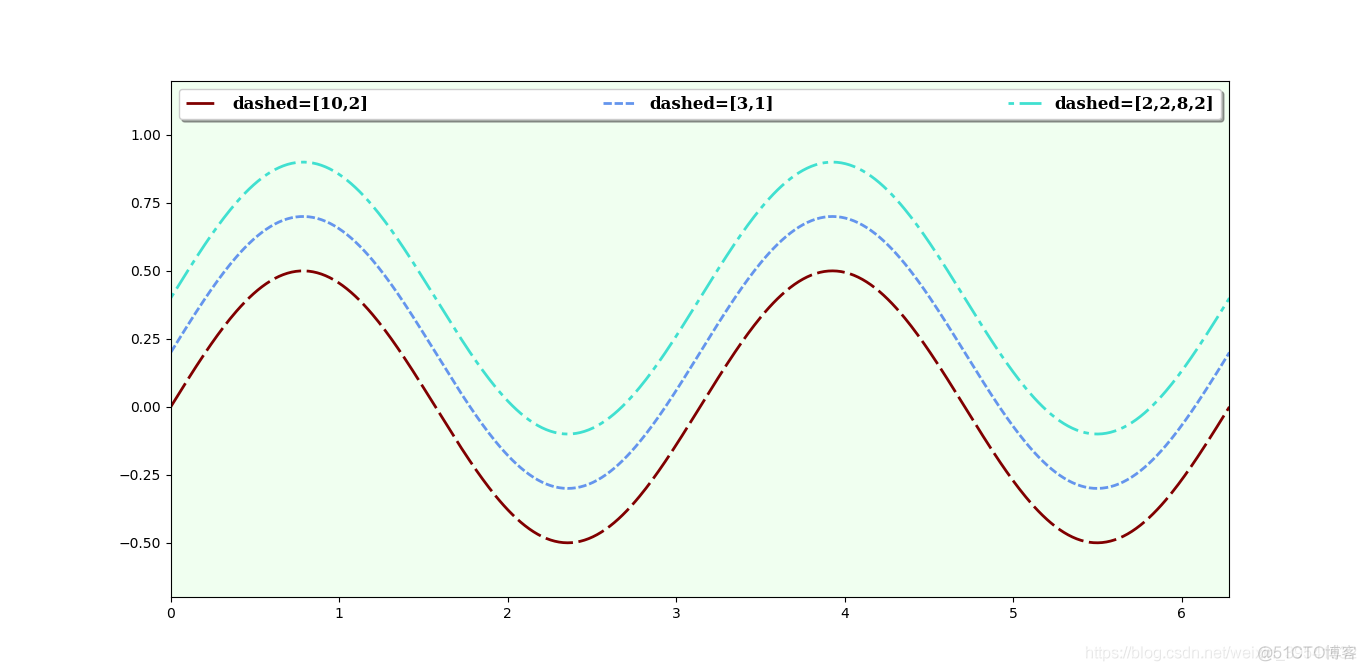
"""
破折号线条样式的不同展现形式的设置方法
:return:
"""
font_style = dict(family="serif", weight="black", size=12)
line_marker_style1 = dict(linestyle='--', linewidth=2, color='maroon',
markersize=10)
line_marker_style2 = dict(linestyle='--', linewidth=2,
color='cornflowerblue', markersize=10)
line_marker_style3 = dict(linestyle='--', linewidth=2, color='turquoise',
markersize=10)
fig = plt.figure()
ax = fig.add_subplot(111, fc='honeydew')
x = np.linspace(0, 2 * np.pi, 500)
y = np.sin(x) * np.cos(x)
ax.plot(x, y, dashes=[10, 2], label='dashed=[10,2]', **line_marker_style1)
ax.plot(x, y + 0.2, dashes=[3, 1], label='dashed=[3,1]',
**line_marker_style2)
ax.plot(x, y + 0.4, dashes=[2, 2, 8, 2], label='dashed=[2,2,8,2]',
**line_marker_style3)
ax.axis([0, 2 * np.pi, -0.7, 1.2])
ax.legend(ncol=3, bbox_to_anchor=(0.00, 0.95, 1.0, 0.05), mode="expand",
fancybox=True, shadow=True, prop=font_style)
plt.savefig(r"E:\Programmer\PYTHON\Matplotlib实践\figure\Figure(Unit "
r"9)\no3.png")
plt.show()
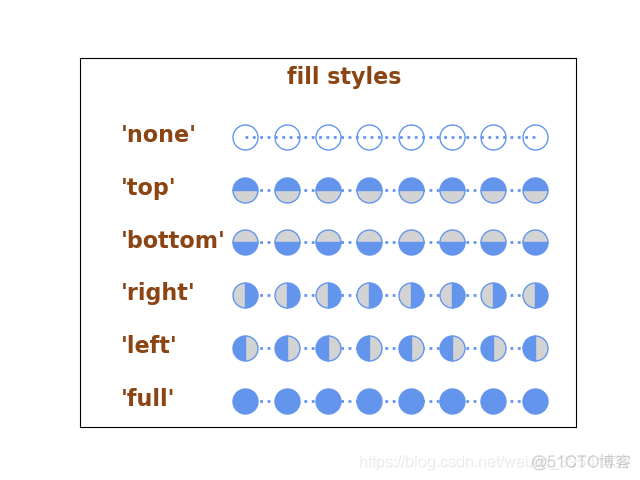
"""
标记填充样式的设置方法
:return:
"""
font_style = dict(family="sans-serif", color="saddlebrown",
weight="semibold", size=16)
line_marker_style = dict(linestyle=":",
linewidth=2,
color='cornflowerblue',
markerfacecoloralt="lightgrey",
marker="o",
markersize=18)
fig = plt.figure()
ax = fig.add_subplot(111)
fillstyleList = ["full", "left", "right", "bottom", "top", "none"]
x = np.arange(3, 11, 1)
y = np.linspace(1, 1, 8)
ax.text(4, 6.5, "fill styles", **font_style)
for i, fs in enumerate(fillstyleList):
ax.text(0, i + 0.4, "'{}'".format(fs), **font_style)
ax.plot(x, (i + 0.5) * y, fillstyle=fs, **line_marker_style)
ax.set_xlim(-1, 11)
ax.set_ylim(0, 7)
ax.margins(0.3)
ax.set_xticks([])
ax.set_yticks([])
plt.savefig(r"E:\Programmer\PYTHON\Matplotlib实践\figure\Figure(Unit "
r"9)\no4.png")
plt.show()
- 本篇博文特别感谢刘大成的《Python数据可视化之matplotlib实践》